Table Of Contents
In this session, we are discussing UNIT-5: ITERATORS & RECURSION | Python Programming important questions with solutions. Hope this session will help you in your upcoming python programming exams.
Dudes 🤔.. You want more useful details regarding this subject. Please keep in mind this as well. Important Questions For Python Programming: *Unit-01 *Unit-02 *Unit-03 *Unit-04 *Unit-05 *Short-Q/Ans *Question-Paper with solution 21-22
Q1. Explain Tower of Hanoi problem in detail OR Explain iterator. Write a program to demonstrate the Tower of Hanoi using function.
Ans.
Iterator:
- An iterator is an object that contains a countable number of values.
- An iterator is an object that can be iterated upon, meaning that we can traverse through all the values.
- Python iterator, implicitly implemented in constructs like for-loops, comprehensions, and python generators.
- Python lists, tuples, dictionary and sets are all examples of in-built iterators.
- These types are iterators because they implement following methods:
- __iter: This method is called on initialization of an iterator. This should return an object that has a next() method.
- next() (or_next_): The iterator next method should return the next value for the iterable. When an iterator is used with a ‘for in’ loop, the for loop implicitly calls next() on the iterator object. This method should raise a StopIteration to signal the end of the iteration.
- For example:
Tower of Hanoi problem :
- Tower of Hanoi is a mathematical puzzle which consists of three rods and a number of disks of different sizes, which can slide onto any rod.
- The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top, thus making a conical shape.
- The objective of the puzzle is to move the entire stack to another rod, obeying the following simple rules:
- Only one disk can be moved at a time.
- Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack.
- A disk can only be moved if it is the uppermost disk on a stack.
- No disk may be placed on top of a smaller disk.
- 3 Dick
Q2.Differentiate between recursion and iteration. OR Discuss and differentiate iterators and recursion. Write a program for recursive Fibonacci series.
Ans.
Property | Recursion | Iteration |
Definition | Function calls itself | A set of instruction repeatedly executed. |
Application | For functions | For Loops |
Termination | Through base case, where there will be no function call | When the termination condition for the iterator ceases to be satisfied |
Usage | Used when code size need to be small and time complexity is not an issue | Used when time complexity needs to be balanced against an expanded code size |
Code size | Smaller code size | Larger code size |
Time complexity | Very high (generally exponential) time complexity | Relatively Lower time complexity (generally polynomial logarithmic) |
Stack | The stack is used to store the set of new local variables and parameters each time the function is called. | Does not use stack |
Overhead | Recursion possesses the overhead of repeated function calls | No overhead of repeated function call |
Speed | Slow in execution | Fast in execution |
Q3.Define recursion. Also, give example.
Answer
- In Python, recursion occurs when a function is defined by itself.
- When a function calls itself, directly or indirectly, then it is called a recursive function and this phenomenon is known as recursion.
- Recursion is the property how we write a function. A function which performs the same task can be written either in a recursive form or in an iterative form.
- Recursion is the process of repeating something self-similar way.
- For example:
Q4. Discuss sorting and merging. Explain different types of sorting with example. Write a Python program for Sieve of Eratosthenes.]
Ans.
Sorting:
- Sorting refers to arranging data in a particular order.
- Most common orders are in numerical or lexicographical order.
- The importance of sorting lies in the fact that data searching can be optimized to a very high level, if data is stored in a sorted manner.
- Sorting is also used to represent data in more readable formats.
Merging:
- Merging is defined as the process of creating a sorted list/array of data items from two other sorted array/list of data items.
- Merge list means to merge two sorted list into one list.
Code for merging two lists and sort it :
a=[ ]
c=[ ]
Different types of sorting are:
- Bubble sort: It is a comparison-based algorithm in which each pair of adjacent elements is compared and the elements are swapped if they are not in order.
- Merge sort:
- Merge sort is a divide and conquer algorithm. It divides input array in two halves, calls itself for the two halves and then merges the two sorted halves.
- The merge() function is used for merging two halves.
- The merge(arr, l, m, r) is key process that assumes that arr[l..m] and arr[m+1..r] are sorted and merges the two sorted sub-arrays into one.
- Selection sort:
- The selection sort algorithm sorts an array by repeatedly finding the smallest element (considering ascending order) from unsorted list and swapping it with the first element of the list.
- The algorithm maintains two sub-arrays in a given array:
- The sub-array which is already sorted.
- Remaining sub-array which is unsorted.
- In every iteration of selection sort, the smallest element from the unsorted sub-array is picked and moved to the sorted sub-array.
- Higher order sort:
- Python also supports higher order functions, meaning that functions can accept other functions as arguments and return functions to the caller.
- Sorting of higher order functions:
- In order to defined non-default sorting in Python, both the sorted() function and .sort() method accept a key argument.
- The value passed to this argument needs to be a function object that returns the sorting key for any item in the list or iterable.
- For example: Consider the given list of tuples, Python will sort by default on the first value in each tuple. In order to sort on a different element from each tuple, a function can be passed that returns that element.
- Insertion sort:
- Insertion sort involves finding the right place for a given element in a sorted list. So in beginning we compare the first two elements and sort them by comparing them.
- Then we pick the third element and find its proper position among the previous two sorted elements.
- This way we gradually go on adding more elements to the already sorted list by putting them in their proper position.
Program:
Q5. Discuss binary search in Python.
Ans.
- Binary search follows a divide and conquer approach. It is faster than linear search but requires that the array be sorted before the algorithm is executed.
- Binary search looks for a particular item by comparing the middle most item of the collection. If a match occurs, then the index of item is returned.
- If the middle item is greater than the item, then the item is searched in the sub-array to the left of the middle item.
- Otherwise, the item is searched for in the sub-array to the right of the middle item.
- This process continues on the sub-array as well until the size of the sub-array reduces to zero.
- Code:
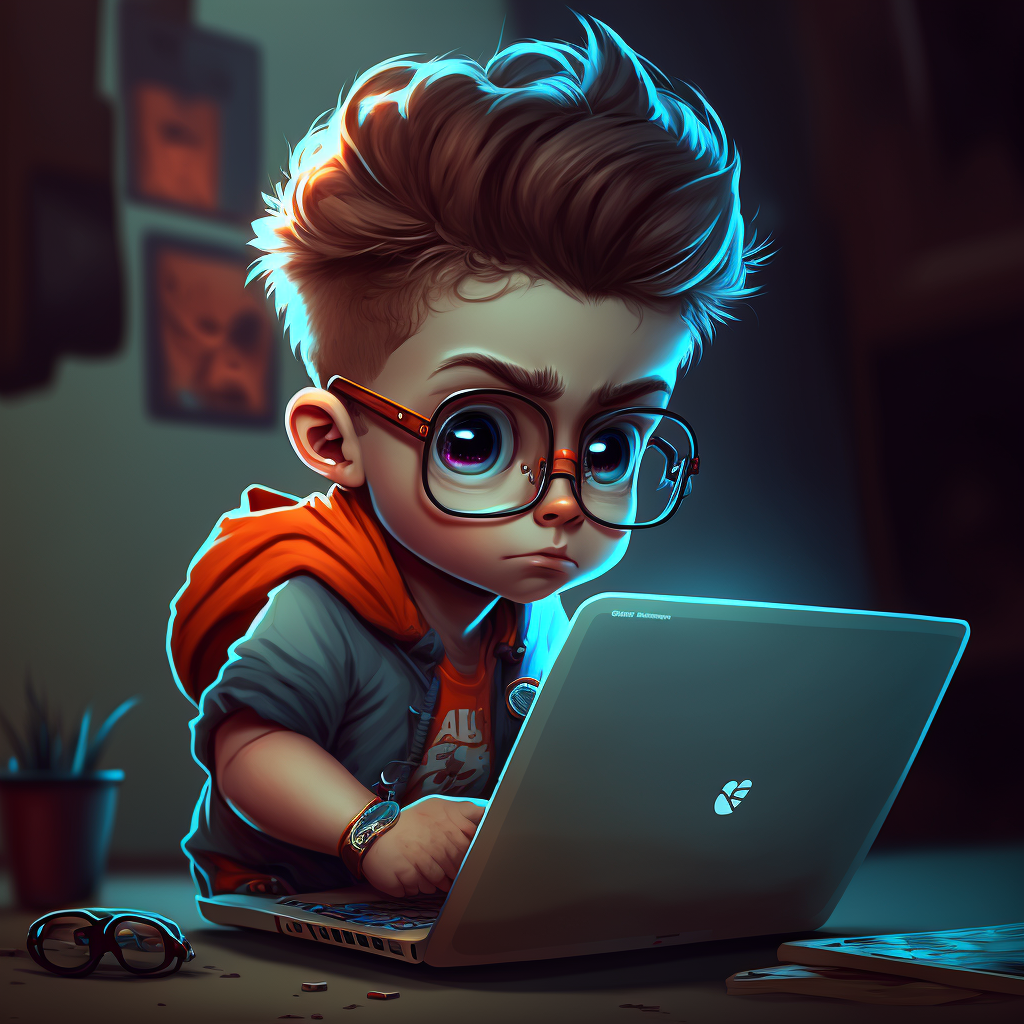
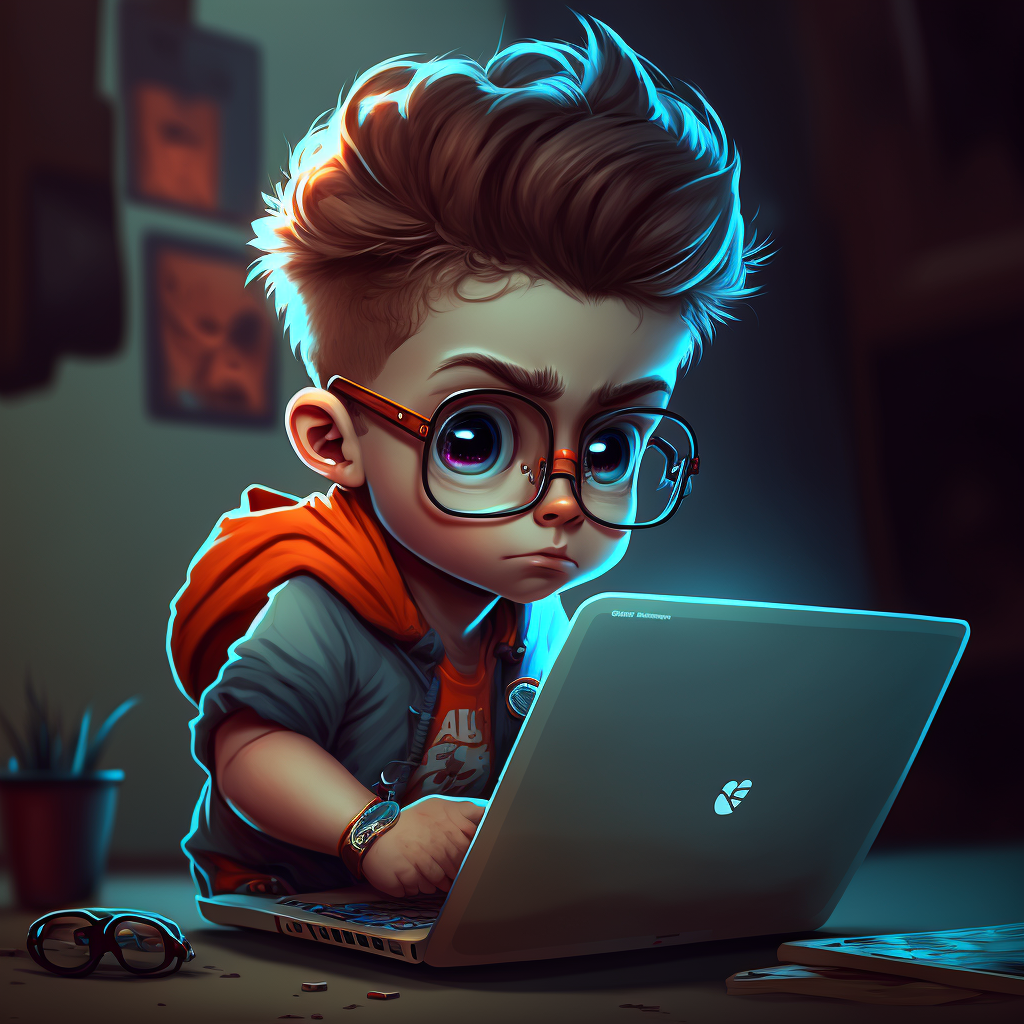
All Question Paper | AKTU Quantum | solution of question paper
Python Programming Important Links:
Label | Link |
---|---|
Subject Syllabus | Syllabus |
Short Questions | Short-question |
Important Unit-1 | Unit-1 |
Important Unit-2 | Unit-2 |
Important Unit-3 | Unit-3 |
Important Unit-4 | Unit-4 |
Important Unit-5 | Unit-5 |
Question paper – 2021-22 | 2021-22 |
AKTU Important Links | Btech Syllabus
Link Name | Links |
---|---|
Btech AKTU Circulars | Links |
Btech AKTU Syllabus | Links |
Btech AKTU Student Dashboard | Student Dashboard |
AKTU RESULT (One VIew) | Student Result |
Important Links-Btech (AKTU) | Python Programming syllabus
Label | Links |
---|---|
Btech Information | Info Link |
Btech CSE | CSE-LINK |
Quantum-Page | Link |
Python Programming Syllabus | Python Programming Syllabus |
4 thoughts on “UNIT-5 : ITERATORS & RECURSION | Python Programming Important Questions”