In this session, we are discussing UNIT-4: SIEVE OF ERATOSTHENES & FILE I/O | Python programming Important questions with solution Hope this session will help you in your python programming exam. so best of luck.
Dudes 🤔.. You want more useful details regarding this subject. Please keep in mind this as well. Important Questions For Python Programming: *Unit-01 *Unit-02 *Unit-03 *Unit-04 *Unit-05 *Short-Q/Ans *Question-Paper with solution 21-22
Q1. Discuss file I/O in Python. How to perform open, read, write, and close into a file? Write a Python program to read a file line-by-line store it into a variable.
Ans.
File I/O:
- A file in a computer is a location for storing some related data.
- It has a specific name.
- The files are used to store data permanently on to a non-volatile memory (such as hard disks).
- As we know, the Random Access Memory (RAM) is a volatile memory type because the data in it is lost when we turn off the computer. Hence, we use files for storing of useful information or data for future reference.
Open, read, write and close into a file:
Open into a file
- Python has a built-in open() function to open files from the directory.
- Two arguments that are mainly needed by the open() function are:
- File name: It contains a string type value containing the name of the file which we want to access.
- Access_mode: The value of access_mode specifies the mode in which we want to open the file, i.e., read, write, append etc.
- Syntax:
close into a file
- When the operations that are to be performed on an opened file are finished, we have to close the file in order to release the resources.
- Python comes with a garbage collector responsible for cleaning up the unreferenced objects from the memory, we must not rely on it to close a file.
- Proper closing of a file frees up the resources held with the file.
- The closing of file is done with a built-in function close().
- Syntax:
write into a file
- After opening a file, we have to perform some operations on the file. Here we will perform the write operation.
- In order to write into a file, we have to open it with w mode or a mode, on any writing-enabling mode.
- We should be careful when using the w mode because in this mode overwriting persists in case the file already exists.
- For example:
The given example creates a file named test.txt if it does not exist, and overwrites into it if it exists. If we open the file, we will find the following content in it.
Output:
Writing to the file line 1
Writing to the file line 2
Writing to the file line 3
Writing to the file line 4
Reading into a file
- In order to read from a file, we must open the file in the reading mode (r mode).
- We can use read (size) method to read the data specified by size.
- If no size is provided, it will end up reading to the end of the file.
- The read() method enables us to read the strings from an opened file.
- Syntax :
file object. read ([size])
For example:
# open the file
Q2. Discuss exceptions and assertions in Python. How to
handle exceptions with try-finally? Explain five built-in exceptions with example?
Ans.
Exception:
- While writing a program, we often end up making some errors. There are many types of error that can occur in a program.
- The error caused by writing an improper syntax is termed syntax error or parsing error; these are also called compile time errors.
- Errors can also occur at runtime and these runtime errors are known as exceptions.
- There are various types of runtime errors in Python.
- For example, when a file we try to open does not exist, we get a FileNotFoundError. When a division by zero happens, we get a ZeroDivisionError. When the module we are trying to import does not exist, we get an ImportError.
- Python creates an exception object for every occurrence of these run- time errors.
- The user must write a piece of code that can handle the error.
- If it is not capable of handling the error, the program prints a trace back to that error along with the details of why the error has occurred.
Assertions:
- An assertion is a sanity-check that we can turn on or turn off when we are done with our testing of the program. An expression is tested, and if the result is false, an exception is raised.
- Assertions are carried out by the assert statement.
- Programmers often place assertions at the start of a function to check for valid input, and after a function call to check for valid output.
- An AssertionError exception is raised if the condition evaluates to false.
- The syntax for assert is: assert Expression [, Arguments]
- If the assertion fails, Python uses ArgumentExpression as the argument for the AssertionError.
Handle exceptions:
- Whenever an exception occurs in Python, it stops the current process and passes it to the calling process until it is handled.
- If there is no piece of code in our program that can handle the exception, then the program will crash.
- For example, assume that a function X calls the function Y, which in turn calls the function Z, and an exception occurs in Z. If this exception is not handled in Z itself, then the exception is passed to Y and then to X. If this exception is not handled, then an error message will be displayed and our program will suddenly halt.
- Try…except:
- Python provides a try statement for handling exceptions.
- An operation in the program that can cause the exception is placed in the try clause while the block of code that handles the exception is placed in the except clause.
- The block of code for handling the exception is written by the user and it is for him to decide which operation he wants to perform after the exception has been identified.
- Syntax:
- Try finally :
- The try statement in Python has an optional finally clause that can be associated with it.
- The statements written in the finally clause will always be executed by the interpreter, whether the try statement raises an exception or not.
- With the try clause, we can use either except or finally, but not both.
- We cannot use the else clause along with the final clause.
- Try…except:
Five built-in exceptions:
- exception LookupError: This is the base class for those exceptions that are raised when a key or index used on a mapping or sequence is invalid or not found. The exceptions raised are:
- KeyError
- IndexError
- TypeError: TypeError is thrown when an operation or function is applied to an object of an inappropriate type.
- exception ArithmeticError: This class is the base class for those built-in exceptions that are raised for various arithmetic errors such as:
- OverflowError
- ZeroDivisionError
- Floating PointError
- For example:
- exception AssertionError: An AssertionError is raised when an assert statement fails.
- exception AttributeError: An AttributeError is raised when an attribute reference or assignment fails such as when a non-existent attribute is referenced.
Q3. Define Class Object with Examples?
Ans.
Class:
- A class can be defined as a blue print or a previously defined structure from which objects are made.
- Classes are defined by the user; the class provides the basic structure for an object.
- It consists of data members and method members that are used by the instances of the class.
- In Python, a class is defined by a keyword Class.
- Syntax: class class_name;
- For example: Fruit is a class, and apple, mango and banana are its objects. Attribute of these objects are color, taste, etc.
In the given example, we have created a class Student that contains two methods: fill_details and print_details. The first method fill_details takes four arguments: self, name, branch and year. The second method print_details takes exactly one argument: self.
Objects:
- An object is an instance of a class that has some attributes and behavior.
- The object behaves according to the class of which it is an object.
- Objects can be used to access the attributes of the class.
- The syntax of creating an object in Python is similar to that for calling a function.
- Syntax :
obj_name = class_name()
For example:
s1 = Student ()
In the given example, Python will create an object s1 of the class student.
Q4. Explain data encapsulation with example.
Ans.
- In Python programming language, encapsulation is a process to restrict the access of data members. This means that the internal details of an object may not be visible from outside of the object definition.
- The members in a class can be assigned in three ways i.e., public, protected and private.
- If the name of a member is preceded by single underscore, it is assigned as a protected member.
- If the name of a member is preceded by double underscore, it is assigned as a private member.
- If the name is not preceded by anything then it is a public member.
Name | Notation | Behavior |
varname | Public | Can be accessed from anywhere |
_varname | Protected | They are like the public member buy they cannot be directly accessed from outside |
__varname | Private | They cannot be seen and accessed from outside the class |
Q5. What do you mean by multiple inheritance? Explain in detail.
Ans.
- In multiple inheritance, a subclass is derived from more than one base class.
- The subclass inherits the properties of all the base classes.
- In Fig. subclass C inherits the properties of two base classes A and B.
- Syntax
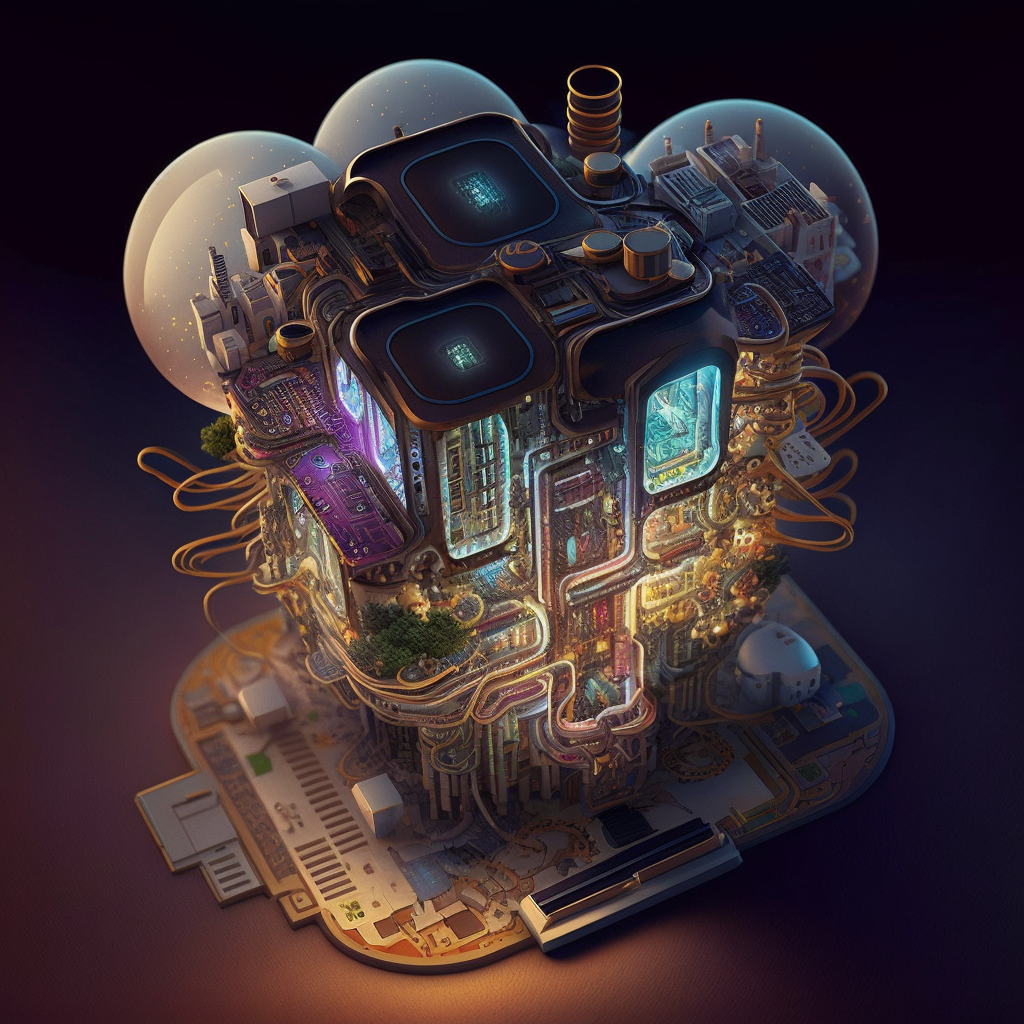
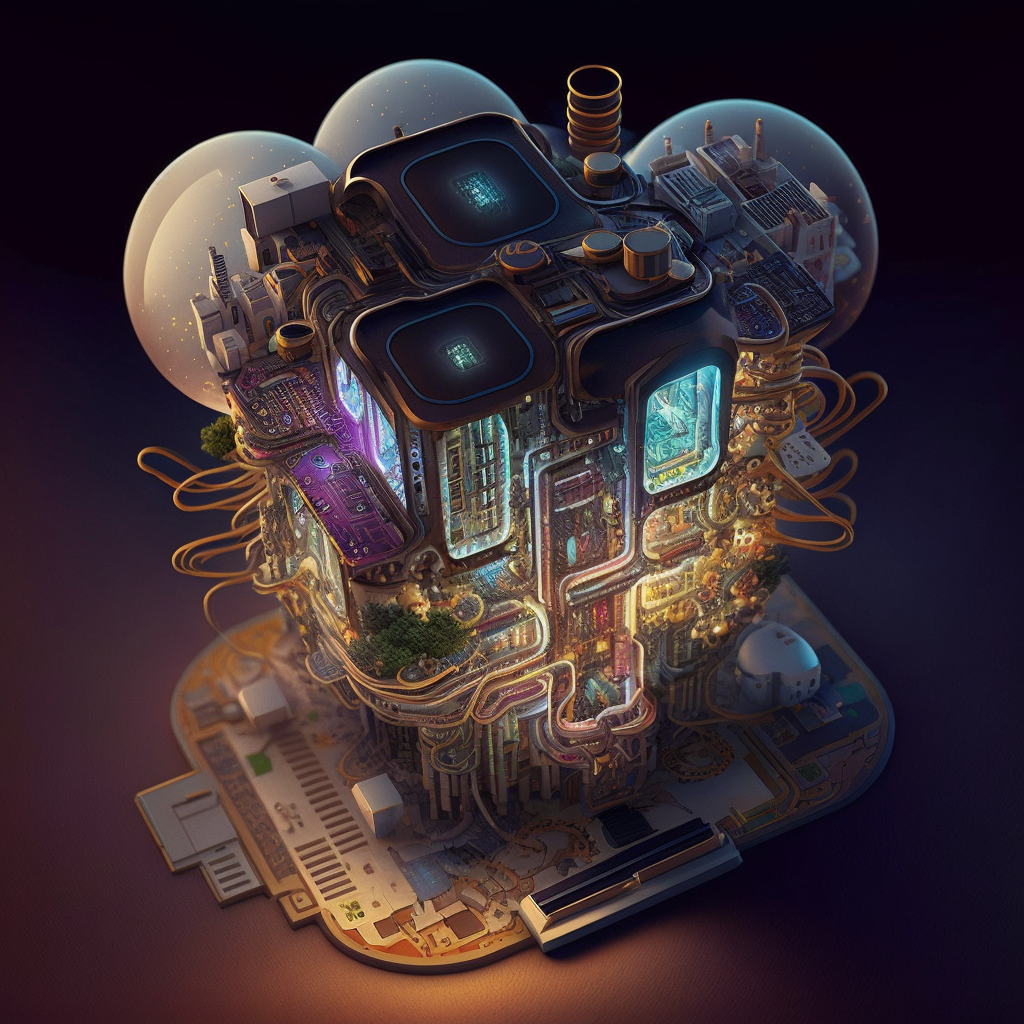
All Question Paper | AKTU Quantum | solution of question paper
Python Programming Important Links:
Label | Link |
---|---|
Subject Syllabus | Syllabus |
Short Questions | Short-question |
Important Unit-1 | Unit-1 |
Important Unit-2 | Unit-2 |
Important Unit-3 | Unit-3 |
Important Unit-4 | Unit-4 |
Important Unit-5 | Unit-5 |
Question paper – 2021-22 | 2021-22 |
AKTU Important Links | Btech Syllabus
Link Name | Links |
---|---|
Btech AKTU Circulars | Links |
Btech AKTU Syllabus | Links |
Btech AKTU Student Dashboard | Student Dashboard |
AKTU RESULT (One VIew) | Student Result |
Important Links-Btech (AKTU) | Python Programming syllabus
Label | Links |
---|---|
Btech Information | Info Link |
Btech CSE | CSE-LINK |
Quantum-Page | Link |
Python Programming Syllabus | Python Programming Syllabus |
4 thoughts on “UNIT-4: SIEVE OF ERATOSTHENES & FILE I/O | Python programming Important questions with solution”